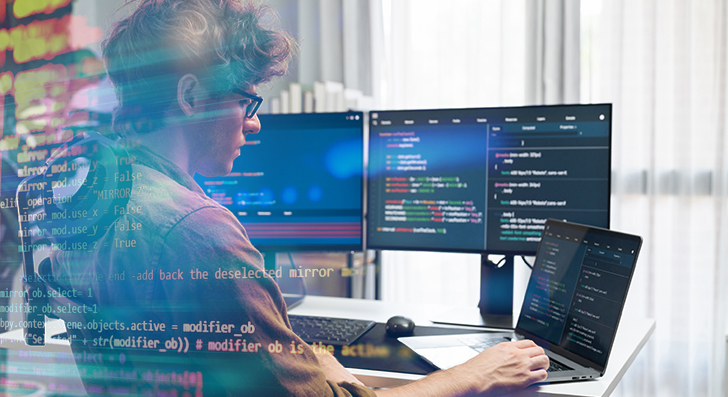
Scalability usually means your application can deal with advancement—additional end users, much more data, plus more website traffic—devoid of breaking. Like a developer, building with scalability in mind will save time and anxiety afterwards. Below’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is just not anything you bolt on later—it ought to be part of your respective strategy from the start. A lot of applications are unsuccessful after they mature quickly for the reason that the initial structure can’t manage the additional load. Being a developer, you have to Consider early regarding how your program will behave stressed.
Start by planning your architecture to be versatile. Steer clear of monolithic codebases the place everything is tightly linked. As a substitute, use modular design or microservices. These designs split your application into smaller, impartial parts. Every single module or assistance can scale on its own with out impacting The full system.
Also, take into consideration your database from working day 1. Will it have to have to handle a million consumers or merely 100? Pick the right sort—relational or NoSQL—determined by how your information will increase. System for sharding, indexing, and backups early, Even when you don’t have to have them yet.
Yet another critical place is to stay away from hardcoding assumptions. Don’t generate code that only operates beneath recent problems. Contemplate what would materialize In the event your person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use structure styles that aid scaling, like information queues or party-pushed programs. These enable your application take care of more requests without getting overloaded.
When you Establish with scalability in your mind, you are not just getting ready for success—you're lessening long term headaches. A perfectly-prepared technique is simpler to maintain, adapt, and mature. It’s superior to get ready early than to rebuild later.
Use the Right Database
Choosing the suitable database is really a key Element of constructing scalable programs. Not all databases are built a similar, and utilizing the Mistaken one can gradual you down as well as trigger failures as your application grows.
Start off by comprehending your details. Could it be highly structured, like rows inside a desk? If Indeed, a relational databases like PostgreSQL or MySQL is an efficient match. These are definitely sturdy with relationships, transactions, and regularity. They also guidance scaling approaches like study replicas, indexing, and partitioning to take care of a lot more traffic and knowledge.
If your facts is more versatile—like person activity logs, product catalogs, or paperwork—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are better at dealing with significant volumes of unstructured or semi-structured information and might scale horizontally much more simply.
Also, consider your browse and create designs. Are you carrying out many reads with fewer writes? Use caching and browse replicas. Will you be handling a large publish load? Look into databases which can deal with substantial produce throughput, or even occasion-based mostly facts storage systems like Apache Kafka (for short-term knowledge streams).
It’s also clever to Believe forward. You might not will need Highly developed scaling attributes now, but selecting a database that supports them implies you gained’t will need to modify afterwards.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your knowledge determined by your obtain styles. And normally observe databases performance when you grow.
In brief, the correct database is determined by your app’s structure, speed needs, and how you expect it to grow. Choose time to select wisely—it’ll save a lot of trouble afterwards.
Improve Code and Queries
Rapidly code is vital to scalability. As your app grows, every compact hold off provides up. Inadequately prepared code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s important to Establish successful logic from the start.
Begin by crafting cleanse, basic code. Stay away from repeating logic and remove something unnecessary. Don’t pick the most sophisticated Answer if a straightforward one particular operates. Keep the features brief, concentrated, and simple to test. Use profiling tools to search out bottlenecks—areas where your code can take also long to operate or utilizes far too much memory.
Following, take a look at your databases queries. These generally slow points down over the code alone. Make certain Each individual query only asks for the info you actually need to have. Avoid Decide on *, which fetches every thing, and instead decide on unique fields. Use indexes to speed up lookups. And prevent performing a lot of joins, Primarily across substantial tables.
If you observe a similar information being requested over and over, use caching. Retail store the outcomes briefly working with tools like Redis or Memcached which means you don’t should repeat expensive operations.
Also, batch your database functions any time you can. As opposed to updating a row one after the other, update them in teams. This cuts down on overhead and will make your app a lot more productive.
Make sure to exam with large datasets. Code and queries that function fantastic with one hundred data might crash whenever they have to manage one million.
To put it briefly, scalable applications are fast apps. Keep your code tight, your queries lean, and use caching when needed. These actions aid your application remain easy and responsive, whilst the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to manage additional buyers plus more traffic. If everything goes through one server, it will quickly turn into a bottleneck. That’s where by load balancing and caching are available. These two applications assistance keep the application quickly, stable, and scalable.
Load balancing spreads incoming visitors across various servers. In lieu of just one server executing every one of the operate, the load balancer routes consumers to diverse servers depending on availability. This means no one server will get overloaded. If a single server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing info temporarily so it can be reused immediately. When end users request a similar data once more—like an item webpage or perhaps a profile—you don’t really need to fetch it through the database anytime. You'll be able to provide it from your cache.
There are 2 common forms of caching:
1. Server-aspect caching (like Redis or Memcached) stores facts in memory for quickly obtain.
2. Customer-side caching (like browser caching or CDN caching) outlets static files near to the user.
Caching lowers database load, enhances speed, and can make your app a lot more economical.
Use caching for things that don’t transform frequently. And generally make certain your cache is up-to-date when data does adjust.
In short, load balancing and caching are straightforward but impressive instruments. Together, they help your application handle a lot more people, stay quickly, and Get well from problems. If you plan to increase, you would like each.
Use Cloud and Container Instruments
To build scalable applications, you would like tools that let your app increase conveniently. That’s where cloud platforms and containers are available in. They provide you overall flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you will need them. You don’t really need to obtain components or guess long run potential. When targeted visitors increases, you can add much more sources with only a few clicks or immediately making use of automobile-scaling. When site visitors drops, you'll be able to scale down to save money.
These platforms more info also present expert services like managed databases, storage, load balancing, and protection instruments. It is possible to target creating your app rather than managing infrastructure.
Containers are another vital Resource. A container deals your app and everything it needs to operate—code, libraries, options—into a single unit. This makes it quick to maneuver your app in between environments, from a laptop computer for the cloud, with out surprises. Docker is the preferred Resource for this.
Whenever your app works by using a number of containers, resources like Kubernetes help you regulate them. Kubernetes handles deployment, scaling, and Restoration. If 1 section of your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate portions of your app into products and services. It is possible to update or scale components independently, which happens to be great for performance and dependability.
In short, working with cloud and container resources suggests you'll be able to scale speedy, deploy quickly, and Recuperate immediately when difficulties materialize. If you'd like your application to develop devoid of limits, start off using these equipment early. They help you save time, decrease possibility, and assist you to keep centered on developing, not repairing.
Watch Every thing
For those who don’t keep track of your software, you received’t know when things go Improper. Checking allows you see how your app is doing, location issues early, and make much better selections as your application grows. It’s a vital part of creating scalable programs.
Get started by monitoring basic metrics like CPU usage, memory, disk Area, and reaction time. These show you how your servers and services are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you obtain and visualize this data.
Don’t just monitor your servers—keep track of your app also. Keep watch over just how long it requires for end users to load web pages, how frequently glitches materialize, and where by they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can help you see what’s happening inside your code.
Create alerts for crucial troubles. For example, if your reaction time goes higher than a Restrict or maybe a assistance goes down, you must get notified quickly. This will help you resolve concerns quick, often before buyers even detect.
Monitoring is additionally helpful when you make variations. When you deploy a completely new element and see a spike in mistakes or slowdowns, you can roll it again ahead of it leads to real problems.
As your app grows, traffic and details enhance. With out checking, you’ll overlook signs of issues until finally it’s too late. But with the appropriate resources set up, you remain on top of things.
In a nutshell, checking will help you maintain your application trustworthy and scalable. It’s not just about spotting failures—it’s about understanding your technique and making sure it really works well, even stressed.
Final Feelings
Scalability isn’t only for huge providers. Even tiny applications want a solid foundation. By planning carefully, optimizing correctly, and utilizing the correct instruments, you are able to Make applications that expand effortlessly with no breaking stressed. Begin modest, think huge, and Make smart.